By: Paul S. Cilwa | Viewed: 7/26/2024 Posted: 8/15/2018 |
Page Views: 842 | |
Topics: #Computers #Projects #Organica | |||
How to implement a browsing history with VB.NET. |
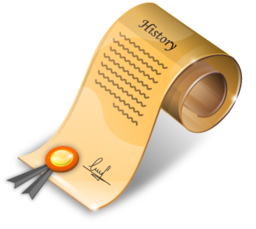
In order to implement our browser's Back and Forward buttons, we must implement a class to store our Document history, and to preserve the current page for a potential Forward when the user clicks the Back button. Fortunately, VB.NET has just the collection class to make short work of this task.
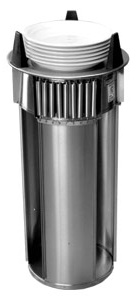
As experienced programmers know, a stack is a special type of collection that remembers the order of objects placed into it; and whatever the last object to be added was, that will be the first to be removed. Think of it like a stack of dishes in a cafeteria dispenser. Items can be pushed onto a stack, or popped off of one.
Here's the commented code of the new module:
History.vb
Public Class History
Public BackPages As New Stack(Of Document)
Public NextPages As New Stack(Of Document)
Public Sub Push(ByRef aDoc As Document)
NextPages.Clear()
BackPages.Push(aDoc)
End Sub
Public Function GoBack(ByRef CurrentDoc As Document) As Document
Dim D As Document
NextPages.Push(CurrentDoc)
D = BackPages.Pop()
Return D
End Function
Public Function GoNext(ByRef CurrentDoc As Document) As Document
Dim D As Document
BackPages.Push(CurrentDoc)
D = NextPages.Pop()
Return D
End Function
End Class
Now, going back to the Frame, we need to add a couple of buttons to the toolbar: One for Back, and one for Forward. I've named them cmd_Back and cmd_Forward, and they both start out with Enabled = False.
We then add an instance of the History class to the Frame.
Frame.vb (enhance)
Public Class Frame
Public Myself As New ThisUser
Public MyDoc As Document
Public MyHistory As New History
Private PageLoaded As Boolean = False
We now need to enhance the Frame's ClickMe procedure to put the current Document into History before switching to the new one:
Frame.vb (enhance)
Public Sub ClickMe(ByVal Pathname As String)
MyHistory.Push(MyDoc)
cmd_GoForward.Enabled = (MyHistory.NextPages.Count > 0)
cmd_GoBack.Enabled = (MyHistory.BackPages.Count > 0)
MyDoc.Find(Pathname).OnClick()
End Sub
Finally, we need to add the command handlers for the Back and Forward buttons.
Frame.vb (append)
Private Sub cmd_GoBack_Click(sender As Object, e As EventArgs) Handles cmd_GoBack.Click
MyDoc = MyHistory.GoBack(MyDoc)
cmd_GoForward.Enabled = (MyHistory.NextPages.Count > 0)
cmd_GoBack.Enabled = (MyHistory.BackPages.Count > 0)
RenderContent()
End Sub
Private Sub cmd_GoForward_Click(sender As Object, e As EventArgs) Handles cmd_GoForward.Click
MyDoc = MyHistory.GoNext(MyDoc)
cmd_GoBack.Enabled = (MyHistory.BackPages.Count > 0)
cmd_GoForward.Enabled = (MyHistory.NextPages.Count >0)
RenderContent()
End Sub
And that's it! Run the app and try clicking on a Folder, then when the new one opens, click the Back button…and then the Forward button. Nifty, no?
In the next chapter we'll implement multiple instances.