By: Paul S. Cilwa | Viewed: 7/26/2024 Posted: 8/9/2018 |
Page Views: 814 | |
Topics: #Computers #Projects #Organica | |||
How to allow user interaction with the WebBrowser control to communicate with VB.NET. |
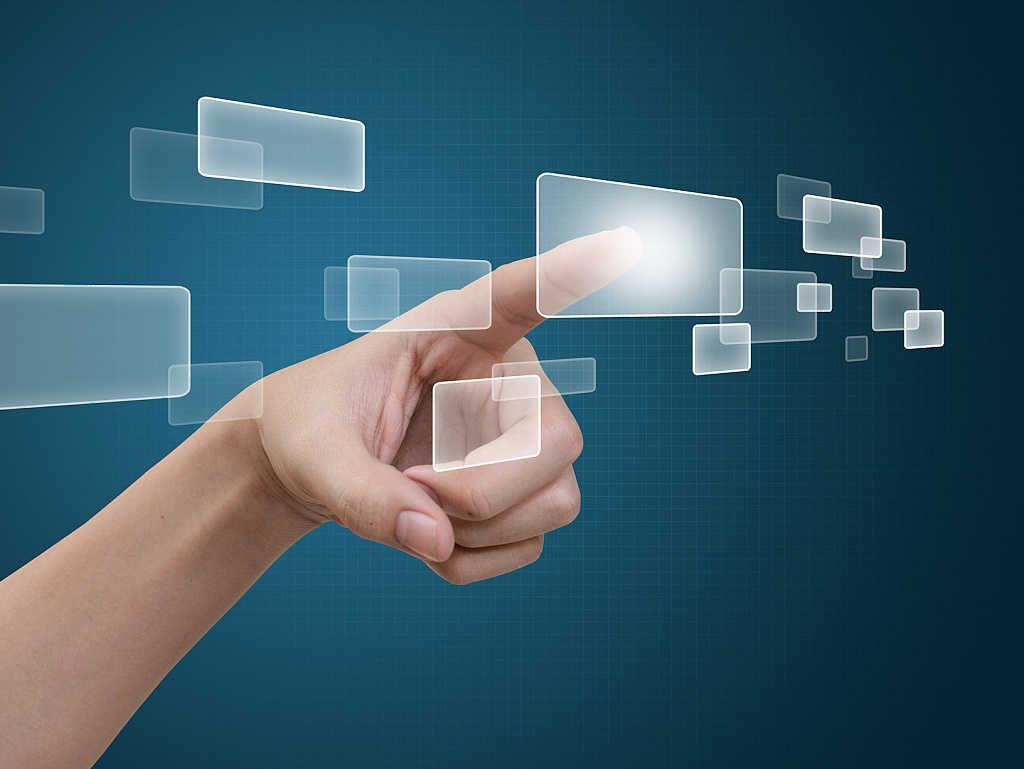
When writing a native Windows application, support of user interaction is a given. But Organica presents itself via HTML and CSS in a web browser window. So, how can we capture user clicks and other interactions so that the underlying VB.NET code can respond? Well, it turns out that doing this is simple, if not very intuitive.
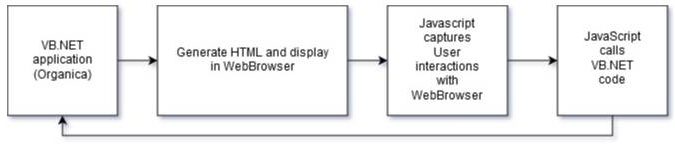
The first thing we must do is tell the system that one of the Organica objects (for example, the Frame) is a trusted COM object. Don't know what that means? Not to worry; you can treat the following as a magic spell:
Frame.vb (insert)
Imports System.Security.Permissions
<PermissionSet(SecurityAction.Demand, Name:="FullTrust")>
<System.Runtime.InteropServices.ComVisibleAttribute(True)>
Public Class Frame
Public Myself As New ThisUser
Private MyDoc As Document
Private PageLoaded As Boolean = False
…
In addition to that, we must add a procedure to Frame that will be invoked when the user does that clicking action. In this procedure, we call the Document's Find function (which we haven't yet written) to locate the child Document that was clicked; we then invoke it's OnClick handler.
Frame.vb (expand)
Public Sub ClickMe(ByVal Pathname As String)
MyDoc.Find(Pathname).OnClick()
End Sub
Now we need to implement the Find and OnClick overridable functions.
Documents.vb FolderDocument (append)
Public Overloads Overrides Function Find(ByVal Key As String) As Document
Return i_DocList.Item(Key)
End Function
Public Overloads Overrides Sub OnClick()
MsgBox("Open " & Pathname, , "Folder")
End Sub
The LinkDocument class also needs an OnClick handler.
Documents.vb LinkDocument (append)
Public Overloads Overrides Sub OnClick()
MsgBox("Open " & Pathname,, "Folder")
End Sub
Now, when we generate the HTML to put into the WebBrowser control, we must include a trigger for the onclick event.
Documents.vb FolderDocument (modify)
Public Overloads Overrides ReadOnly Property RenderHeader As String
Get
Dim Buffer As String
Buffer = "class='organica_Document organica_Folder'
ConCat(Buffer, "onclick='window.external.ClickMe(""" & ")
ConCat(Buffer, "PathName.Replace("\", "\\") & """)'>"")
ConCat(Buffer, "<img src='" & IconPath & "'><div>")
ConCat(Buffer, "<h1>" & Filename & "</h1>")
ConCat(Buffer, "<p>" & Pathname & "</p>")
ConCat(Buffer, "</div></div>")
Return Buffer
End Get
End Property
Documents.vb LinkDocument (modify)
Public Overloads Overrides ReadOnly Property RenderHeader As String
Get
Dim Buffer As String
Buffer = "<div class='organica_Document organica_Folder' "
ConCat(Buffer, "onclick ='window.external.ClickMe(""")
ConCat(Buffer, Pathname.Replace("\", "\\") & """)'>")
ConCat(Buffer, "<img src='" & IconPath & "'><div>")
ConCat(Buffer, "<h1>" & Filename & "</h1>")
ConCat(Buffer, "<p>" & Pathname & "</p>")
ConCat(Buffer, "</div></div>")
Return Buffer
End Get
End Property
Note that, instead of a JavaScript function, we invoke window.external.ClickMe as the target of the onclick event.
So now, when you run Organica and click on one of the real or virtual folders it lists, you'll see a message box like this one, proving that the connection between VB.NET, the WebBrowser, JavaScript and back to VB.NET is complete and functional.
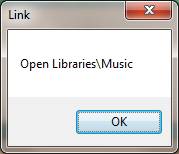
Now, all we have to do is enhance the FolderDocument stub to allow our test user to click on a Folder and "open" it.
Documents.vb (enhance)
Public Overloads Overrides Sub OnClick()
Frame.MyDoc = Me
Frame.RenderContent()
End Sub
Don't forget to make the above change to the LinkDocument class, too.
To make things more interesting, I went ahead and added a few Folders to the Contacts Folder, so we'd have something to see. So, when I click on Contacts, it loads:
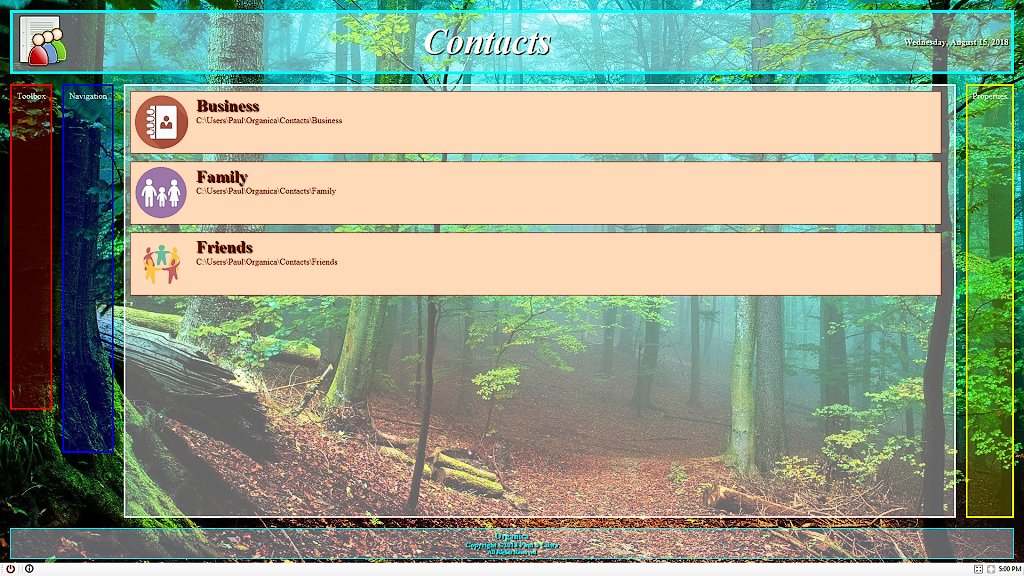
Moreover, if you click on one of the new Folders, it will load as well. Unfortunately, there's nothing in them, so nothing will show up in the organica_Content element. But the caption and icon will definitely change.