By: Paul S. Cilwa | Viewed: 7/27/2024 Posted: 6/11/2018 |
Page Views: 883 | |
Topics: #Computers #Programming #Organica #VB.NET #HTML #DOM | |||
How to build into Organica the ability to create pages to order from a script. |
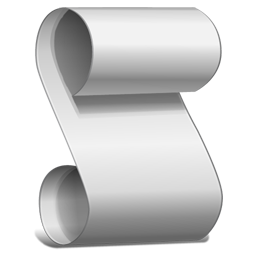
Previously we created an HTML file that can be viewed in any browser window. Then, we designed a browser of our own for viewing it. But since the web page (currently) has no links, our browser can't go anywhere or do anything else.
What we want is to use the web page as a template, which can then be modified by the Organica browser as needed, before a page is ever shown.
There are primarily two ways of accomplishing this. The first is to load the page, then use methods of the WebBrowser control to modify sections as needed. The second is to read the HTML file, modify it, and load it directly into the control.
I find the first technique to be the simpler of the two—especially since we already provided an ID attribute to the elements we'd like to modify.
Be aware, though that you cannot modify the document until after it's been completely loaded. The only truly reliable way to do this is to handle the WebBrowser control's DocumentCompleted event. Which, in point of fact, makes it the perfect place to put the modification code. Here's a quickie text example:
Frame.vb
Private Sub Canvas_DocumentCompleted(sender As Object, _
e As WebBrowserDocumentCompletedEventArgs) _
Handles Canvas.DocumentCompleted
Canvas.Document.GetElementById("organica_Icon").SetAttribute _
("src", Myself.PhotoPath)
Canvas.Document.GetElementById("organica_Nickname").InnerText = _
Myself.Nickname
Canvas.Document.GetElementById("organica_Date").InnerText = _
DateTime.Now.ToString("D")
End Sub
Start the app. Now the banner, instead of displaying the words "Welcome to Organica", you now get to read your username and see your picture.
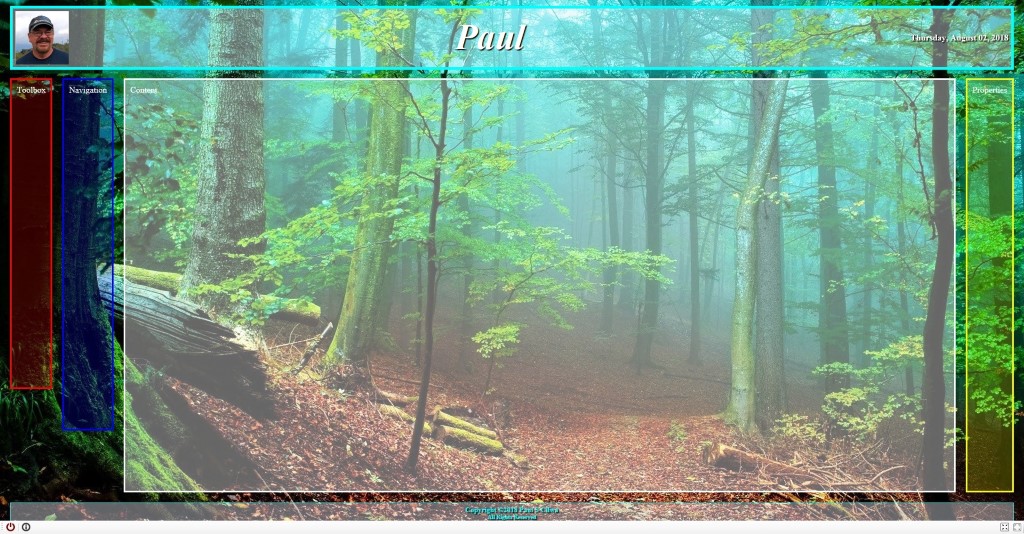
When the first instance of Organica starts, we want its "current directory" to be the user's account folder. This is the one, under the Users folder, with your name on it. It contains subfolders such as My Music, My Videos, Desktop, and so on. However, additional copies of Organica, which will be started to open a specific document other than the user root should be the directory of the desired document. Those additional copies should not be full-screen, either.
Windows apps (such as Organica) are permitted to be started with arguments or parameters, and there's a system function that returns them. The first argument is the pathname of the application executable. If that's the only argument, we know this is the first copy of Organica and should be positioned to the user directory. But if there's a second, we assume that to be the name of the folder or file that contains the document the user wants.
To make all this happen, we need a new procedure in Frame that will examine the argument list and return the correct directory.
Frame.vb
Private Function StartDirectory()
Dim A As String() = Environment.GetCommandLineArgs()
If A.Count > 1 Then
Return A(1)
Else
Return Myself.Directory
End If
End Function
We then call the new function from the Frame's New procedure:
Frame.vb
Public Sub New()
PresetRegistryValues()
InitializeComponent()
ChDir(StartDirectory)
If Environment.GetCommandLineArgs().Count <= 1 Then
GoFullScreen(True)
End If
End Sub
Next: We'll start examining the contents of the start directory!