By: Paul S. Cilwa | Viewed: 7/26/2024 Posted: 6/11/2018 |
Page Views: 1159 | |
Topics: #Computers #Programming #Organica #VB.NET | |||
How to get the current user account's info with VB.NET. |
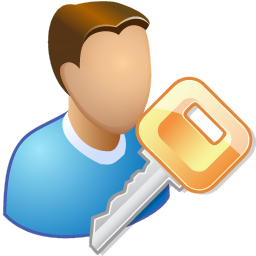
The next step in creating our File Explorer replacement (Organica) is to automatically move to the folder of the currently logged-in user.
But, wait—how can we find out where that is?
In fact, there's a lot of user account information to which we might like access. And that's what the ThisUser class will be for.
So, in our Organica project, add a new class module called "ThisUser" and enter the following code:
Creating the Class
The built-in WindowsIdentity class already encapsulates some pieces of the information we want.
ThisUser.vb
Imports System.Security.Principal
Public Class ThisUser
Public KnownFolders As KnownFolders
Private i_Nickname As String
Private Myself As WindowsIdentity
Public Sub New()
Myself = System.Security.Principal.WindowsIdentity.GetCurrent()
i_Nickname = Myself.Name.ToString
Dim i As Int16
i = i_Nickname .IndexOf("\")
If i > 0 Then
i_Nickname = i_Nickname .Substring(i + 1)
End If
Photo = Image.FromFile(GetPhotoPath())
End Sub
Public ReadOnly Property Nickname As String
Get
Return i_Nickname
End Get
End Property
End Class
The class' New() subroutine sets the WindowsIdentity object and then extracts the username from it. However, this username is the network username, which includes the computer's name. For example, on my development computer, my full username is "RENDERING\Paul". But this isn't quite what I want, because the name of the user (in this case, Paul) is all I need as long as I continue to hunt for folders on the same computer. So the piece of code that follows the assignment to i_Nickname strips off the computer identifier, leaving me with just "Paul".
The user Nickname cannot be changed by this app, so the internal property i_Nickname is exposed through the ReadOnly property code Nickname.
Accessing the User Photo
The Photo property is to return the photo the user has selected to represent themself. Now, this photo is the very devil to locate. Obviously the system knows where it is, to use it on the Windows menu and other places it appears. But the system seems to extract it as needed, and then places the bitmap in a temp folder in the hidden User\AppData folder.
Again, in my case, the file will be named "Paul.bmp". The following function will calculate and return the full pathname of the photo file.
ThisUser.vb
Public ReadOnly Property PhotoPath As String
Get
Dim BasePath As String
BasePath = Environment.GetFolderPath( _
Environment.SpecialFolder.LocalApplicationData) & "\Temp\"
Return BasePath & Nickname & ".bmp"
End Get
End Property
Which then makes it simple to return an actual image from the photo, in case we should need to do that.
ThisUser.vb
Public ReadOnly Property Photo() As Image
Get
Return Image.FromFile(PhotoPath)
End Get
End Property
Testing the Class
The easiest way to test the class is to add an About box to the Organica app, and use it to display the information we've obtained. We can add a Windows Form, name it About, and place a PictureBox control and a Label control on it, as well as the obligatory OK button.
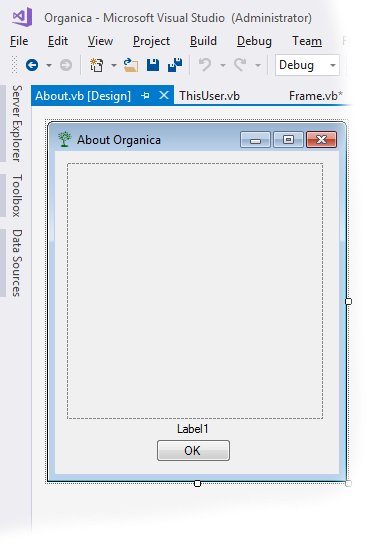
Then we must add another button to the ToolStrip to make the About box appear.
Frame.vb
Private Sub cmd_About_Click(sender As Object, e As EventArgs) _
Handles cmd_About.Click
About.ShowDialog()
End Sub
Then the following code will make it work:
About.vb
Public Class About
Private Sub About_Load(sender As Object, e As EventArgs) _
Handles MyBase.Load
Dim U As New ThisUser
PictureBox1.Image = U.Photo
Label1.Text = U.Nickname
End Sub
Private Sub cmd_OK_Click(sender As Object, e As EventArgs) _
Handles cmd_OK.Click
Me.Close()
End Sub
End Class
Start the app and click the About button to see the result. (Your result should be your photo and name, not mine!)
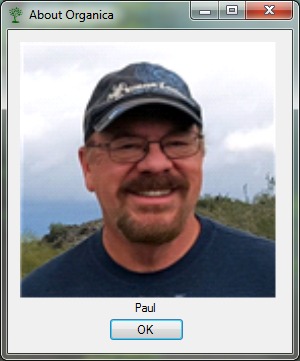
User Profile Directory
Our last (for now) addition to the ThisUser class is to provide a read-only property that returns the official user directory. That's the subdirectory under Users with your name. (Mine is "c:\Users\Paul".)
ThisUser.vb
Public ReadOnly Property Directory() As String
Get
Return Environment.GetFolderPath(Environment.SpecialFolder.UserProfile)
End Get
End Property
We need only add two lines to the Frame code, to "position" the running application to the user directory.
ThisUser.vb
Public Myself As New ThisUser
Public Sub New()
PresetRegistryValues()
InitializeComponent()
ChDir(Myself.Directory)
GoFullScreen(True)
End Sub