By: Paul S. Cilwa | Viewed: 5/9/2024 Posted: 2/24/2022 |
Page Views: 727 | |
Topics: #Organica #VisualBasic #VB.NET #ClassLibrary #OrganicaLib | |||
Easily store information in the System Registry. |
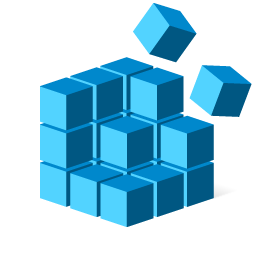
In Windows, the System Registry holds setting information for Windows itself, as well as for any number of applications. Visual Basic used to provide a simple means of reading to and writing from the System Registry, but then they split .NET into several branches, in an effort to promote programming for any generic platform, not just Windows. So now, applications that are .NET CORE-based and have code using those methods is broken. So I've replaced them and put them in a new class, SystemRegistry, added to OrganicaLib.
This is a simple project, using the original Win32 functions as encapsulated in CORE's Registry class. Also, we'll restrict our use of the Registry to the Current User, the only area that doesn't require Administrator permission. (That's virtual anything you'd want to write, anyway, unless you literally want to write a replacement for RegEdit.)
To follow along, add a class module to your OrganicaLib project and give it the name, "SystemRegistry". This class, which must import System.Win32, has just four components: New(); a Value property that can be read or written to; and three cleanup procedures, DeleteValue, DeleteApp, and DeleteCompany.
To use, just create an instance of the SystemRegistry object, providing a name for the app and, optionally, a company name. For example:
Imports OrganicaLib
Class MyClass
Public CurrentNumber as UInt16 = 0
Public Sub GetValue()
Dim AppRegistry As New SystemRegistry("Arrowverse")
V = AppRegistry.Value("CurrentNumber")
If V > 0 Then
CurrentNumber.Value = V
End If
End Sub
End Class
So, now that you have an idea how to use it, let's see how to write it.
Imports Microsoft.Win32
Public Class SystemRegistry
Private BaseKey As RegistryKey = Registry.CurrentUser.OpenSubKey("Software", True)
Private CompanyKey As RegistryKey
Private AppKey As RegistryKey
Public Sub New(MyApplication As String, Optional MyCompany As String = "")
Dim K As RegistryKey
K = BaseKey
If MyCompany > "" Then
CompanyKey = K.CreateSubKey(MyCompany, True)
K = CompanyKey
End If
AppKey = K.CreateSubKey(MyApplication, True)
End Sub
…
As you can see, the class has three private properties to help it do its job; each is of the RegistryKey class. Registry entries use the node system of traversing and accessing values. So the top node is the BaseKey; if a company name is provided, the CompanyKey will point to that; and, regardless, the AppKey stores to the values for the current application.
…
Public Property Value(KeyName As String) As String
Get
Return AppKey.GetValue(KeyName)
End Get
Set(aValue As String)
AppKey.SetValue(KeyName, aValue)
End Set
End Property
…
The Value property encapsulates the GetValue and SetValue functions. The desired key is specified as an index to the object, as seen in the example.
The DeleteValue method exists to do just that. The DeleteApp and DeleteCompany methods are intended for uninstall operations.
…
Public Sub DeleteValue(KeyName As String)
AppKey.DeleteValue(KeyName)
End Sub
Public Sub DeleteApp()
BaseKey.DeleteSubKey(AppKey.ToString)
End Sub
Public Sub DeleteCompany()
If CompanyKey IsNot Nothing Then
BaseKey.DeleteSubKeyTree(CompanyKey.ToString)
End If
End Sub
End Class
And that's all there is to today's project!