By: Paul S. Cilwa | Viewed: 5/18/2024 |
Page Views: 703 | |
Topics: #VisualBasic6.0 #ProgrammingforMicrosoftWindows | |||
Chapter 5 of this free online course in Visual Basic 6.0. |
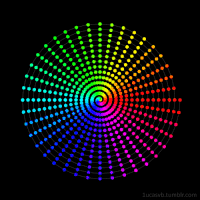
In Windows, colors are represented (as is everything else) by numbers—specifically, 24-bit numbers. The 24 bits are divided into three sets of 8 bit values, one each for red, green, and blue intensities. As it turns out, virtually any color can be represented as combinations of red, green and blue.
Now, if you've been paying attention, you know that there are 16-bit wide numbers in Visual Basic (Integers) and 32-bit wide numbers (Longs) but no 24-bit numbers. So, how do they do this?
Simply: They use Longs, and reserve the extra 8 bits. If you are programming in C or C++ for windows, there is a special reserved name for the data type that holds colors: It's called a COLORREF.
There is a major weakness in the concept of a COLORREF: It is only good for "hard-coded" colors, that is, colors chosen by the programmer. But most of the colors you see on a person's screen were chosen by that person, in Control Panel. And, in the Windows API, there is a completely different method of obtaining those user-selected colors for use as COLORREFs.
By the time Alan Cooper got around to inventing Visual Basic, it was clear that one common method of working with colors was needed. And, since that extra 8-bits was available, he decided to use it. If those 8 bits contain a zero (as they do in all COLORREFs) the value is treated as a perfectly ordinary COLORREF. If they contain a 1, the remaining 24 bits is treated as an index into the set of user-defined colors. And, if it contains a 2, the remaining 24 bits is treated as a palette reference (which we'll explore shortly).
Since the 32 bits are now used differently, they should be stored in a data type of a unique name (even though it's really just a Long). The name that was chosen, perhaps too hastily, was OLE_COLOR. ("OLE" is an acronym that means "object linking and embedding", and has absolutely nothing to do with color; but for a couple of years Microsoft stuck it in front of just about everything.)
Here's the internal format of an OLE_COLOR:
0 | Blue | Green | Red |
---|
Now, as a general rule, you don't want to actually change colors, even though it's easy to do in Visual Basic. Users have a wide range of visual capabilities, challenges, and preferences; and when they choose a color in Control Panel it's generally for a reason. Especially, you should not take it upon yourself to redecorate an entire application, even though you can. There are a few exceptions:
- Card games traditionally play on a form window with a green background, to create the illusion that the user is actually in Las Vegas.
- Game programs of other types often don't look like Windows programs at all; you can do whatever to them you wish.
Forms and many controls and other objects, have a pair of color-related properties these are ForeColor and BackColor. BackColor is usually the background color of the object; ForeColor is the color to be used for text or lines or other things drawn on the surface of the object. Since these two colors work as a pair, it's particularly important that you do not change one of them and not the other. For example, if you change ForeColor to red and leave BackColor to a user-selected hue, there's no guarantee that some user, somewhere, might not sometime set that hue to red, causing your red letters against his or her red background to result in illegible text.
Other commonly-used properties are FillColor and MaskColor, which we'll examine shortly.
Working with Graphics
The Picture property, which Forms, several controls and other objects have, is used to store a graphic to be seen by the end user at run-time.
Visual Basic objects can support three different types of graphic:
- Bitmaps
- Icons
- Metafiles
The usual way to set the Picture property is by clicking on the "…" button on the property page, and selecting an appropriate graphic file. If you have a picture into the Clipboard, you can also select the object and paste; Visual Basic is smart enough to know that if you are pasting a picture, and the currently selected object has a Picture property, you must want to set that property with the pasted graphic.
If you want to remove a picture at design-time, select the property and tap the Delete key.
Picture properties can also be set at run-time:
MyForm.Picture = LoadPicture("MyPicture.bmp")
or
MyForm.Picture = MyOtherForm.Picture
To remove a picture at run-time without replacing it, use
MyForm.Picture = LoadPicture
Bitmaps
A bitmap is just what you think: a two-dimensional array of small boxes, each with a color value. Cross-stitch embroidery is a bitmap. So is your TV screen, and so is this monitor.
The size of a bitmap—which is significant since it affects the amount of memory required to display, and the size of your executable file if it is loaded at runtime—depends not only on its two-dimensional size, but on its color depth. For example, while a monochrome bitmap only requires one bit per pixel (pixel = picture element), a sixteen-color bitmap requires four, and a full-color (photographic) bitmap requires twenty-four! That means that a full-screen, 800 * 600 pixel, full-color bitmap, requires about 1.3 mb of storage!
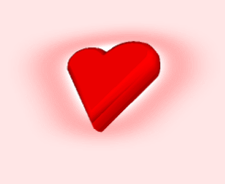
16,000,000 colors; 240 kb
Now, there are ways of managing that. One is to fake a full-color bitmap by figuring out how many colors are actually required. For example, here is a full-color graphic of a valentine heart:
As pretty as it looks, it doesn't actually use all 16,000,000 colors a full bitmap can display. In fact, it only uses 3,670 of them. Now, there is no bitmap format that only displays 3,670 colors. But is there a way we can reduce the storage requirements of this graphic?
One way is to cheat by using a scheme that uses fewer colors—but states which colors, out of the full 16,000,000, those colors are to be. They can be any shade, however exotic. That's called using a palette. Most graphics programs will create a palette for you, automatically, by choosing the most commonly used colors in the graphic and dithering the in-between shades to fake the rest.

Here's our heart graphic reduced to just 256 colors. You can see that the glow and shading around the heart is slightly less subtle than in the full-color version, but not objectionably so. Yet, as a bitmap, the second version takes one-third the space of the first!
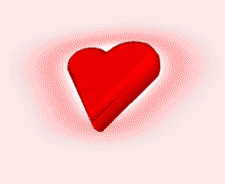
Finally, let's look at the same graphic diminished to just sixteen different shades of red—with dithering. As you can see, the results are inferior to those of the fuller-color versions; yet the idea remains and, in some cases, reducing to sixteen colors—especially in the case of a cartoonish-like figure—might result in no visible deterioration of the image at all.
Bitmaps can be created via a wide variety of tools. Basically, any image editor with the word "paint" in its title is likely to create bitmaps, but many other top-end image editors (like Microsoft PhotoDraw 2000 version 2, and Adobe Illustrator) can produce bitmaps as well.
Alternate Bitmap Formats
With the advent of the Internet, attempts have been made to come up with alternate ways of storing bitmaps that wouldn't take up so much space—and would, therefore, take less time to transmit over a phone line.
You can read more about these and many other graphics file formats at http://www.dcs.ed.ac.uk/home/mxr/gfx/2d-hi.html.
JPEG
JPEGs (extension .jpg) are a compressed, "lossy" format for storing photo-quality images. "Compressed" means they take up far less space than a bitmap; "lossy" means this is accomplished by not storing every single bit. If you repeatedly load and save a JPEG image, it will appear to become more and more "washed out", like a copy of a copy. However, the degree of lossiness is controllable; you can choose lossiness over size to obtain the smallest possible file without losing so much quality that the image becomes unusable.
JPEG is short for the Joint Photographic Experts Group. This is a group of experts nominated by national standards bodies and major companies to work to produce standards for continuous tone image coding.
JPEG, the people, is not the same as JPEG, the standard. In fact, the standard isn't correctly known as JPEG at all—it's actually called JFIF (JPEG File Interchange Format)! However, most people call it JPEG and we will do that here.
JPEGs are always stored with a palette of 16,000,000 colors; but they have the attribute of being expanded into however many colors are available. In other words, if you display a full-color JPEG on a monitor that can only display 16 colors, the JPEG expander will automatically dither so that the result is the best possible for the display. Bitmaps, on the other hand, look ghastly when viewed in fewer colors than they were designed for.
Compression vs. Size
Below you will see three versions of our heart graphic at different levels of compression: 10%, 20%, and 80%. Remember, the more compression, the more loss.
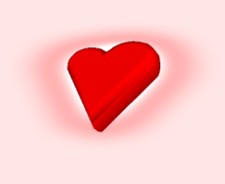
10% compression; 4.62 kb
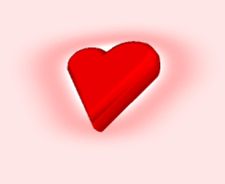
20% compression; 3.4 kb

80% compression; 1.92 kb
GIF
Before everyone was on the Web, there was CompuServe, which made use of the LZW compression algorithm to form the Graphics Interchange Format. Although CompuServe at first claimed exclusive proprietorship of this format, the LZW people took them to court; and now the official position, in the words of CompuServe's vice president, is that "CompuServe is committed to keeping the GIF 89A specification as an open, fully-supported, non-proprietary specification for the entire on-line community including the World-Wide Web."
The GIF specification uses only a 256 color palette, so it is better for drawings and cartoons than most photographs. It is compressed, but not lossy. Its main feature, though, is that GIFs can include a transparency channel, that is, an extra bitmap that indicates which cells are to be transparent and which will come from the graphic, itself. The transparency channel is just one bit wide; so a given cell is either transparent or it is not. That allows for limited subtlety in the graphic.
Here's our heart graphic as a GIF with transparent areas:
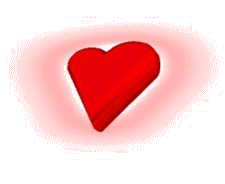
GIF with transparency channel; 9.75 kb
MaskColor
Since most bitmap formats do not include a transparency channel, some objects and controls allow you to specify a color that will be treated as a transparent area. After all, with 16,000,000, surely you can spare one! Whatever color you assign to the MaskColor property, will be used to identify cells of the bitmap that will be transparent.
Icons

Windows 1.0 was written long before people were hurling graphics around on the Internet; and its designers perceived three special needs early on: How to deliver small graphics to the screen on a wide variety of display adapters, how to do it quickly on the slow machines of the period, and how to allow those graphics to be of irregular shapes—that is, how to provide a transparency channel with the graphic.
The icon format dealt with all these problems via several mechanisms:
A transparency channel accompanying each image allows three "colors": Use the bitmap, make the pixel transparent, or invert the underlying pixel.
Each icon file can hold several images, each optimized for specific hardware, like monochrome graphics cards, CGA, EGA, and so on.
The decision as to which image to use is made by Windows, based on its knowledge of the hardware; and the images are small, so they are quickly transferred to the screen.
Nowadays, of course, we don't have a wide range of video hardware—it's pretty much all VGA (or "Super VGA", which is an extension of the same thing). However, with Windows 95, the concept of "small" (16 * 16 pixel) icons was introduced; and the icon format easily supports that option, as well.
Visual Basic 6.0 comes bundled with IconDraw, an extremely elementary and yet capable icon editor. If you've bought Visual Basic as part of Visual Studio, you also have access to the icon editor functions in Visual Studio's editor, which is much better. There are also inexpensive and free third-party icon editors available for download from the Web.
Metafiles
The last class of graphic is different, because it is not a bitmap—although it may contain one or more bitmaps. The "metafile", also called a "Picture" some places in Windows, is not a drawing—it is instructions for a drawing, such as, "Put a red disk with a green outline here; put an orange square over there and write this text, in this font, over the entire thing."
Metafiles automatically draw themselves into the available space.
Although Microsoft doesn't provide any metafile editors of their own, they are willing to supply plenty of metafiles: Many, if not most, of the clip art in Clip Art Gallery (bundled with Microsoft Office) are metafiles. If you really want to make your own, there are any number of free or shareware metafile editors available on the Web; any image editor with "Draw" in its name probably creates metafiles.
The Icon Property
Every form has an additional property that holds the icon that represents that form. It is the Icon property, and it is like the Picture property in usage except that it can hold only icon images (not bitmaps or metafiles).
Even if you do not assign a value to this property, there is a default icon for each form.
When compiled, the entire application is represented by just one icon from the whole project. That icon must be "in" the set icon for one of the project's forms—usually, the startup form. However, it can be set via the Project..Properties command, on the Make tab.